Git – Basics


What is Git?


How to Install Git?
For Windows – Windows Download Link
For Mac – Mac Download Link
For Linux – Type the following commands from the Shell.
$ sudo apt-get update
$ sudo apt-get install git
After successful installation of Git, you can configure your user name and e-mail ID using the following commands.
$ git config --global user.name "First Last"
$ git config --global user.email "myemail@domain.com"


Git Terminologies
Before starting with the basics, let’s explore a few terminologies:
-
Git Repository: A directory with
.git
folder, where all the contents are tracked for changes. -
Remote: It refers to a server where the project code is present. For example, Github and Gitlab.
-
Commit: It is similar to version. When you make changes in a file, you need to commit the changes in order to save and create its new version, which will create a unique commit hash (like version number).
-
Origin: It is a variable where Git stores the URL of your remote repository. For example, origin => www.github.com/username/myrepo


Git Basic Commands
-
git init – adds
.git
folder and initializes the current folder to track its changes -
git status – displays the current state of the staging area and the working directory, that is, which files are added/removed/modified
-
git diff shows the exact changes with line and column number
-
git add adds the changes to the staging area. If you have added a new file, this command starts tracking the file for modifications.
-
git commit will save all the changes with a unique hash number in the local repository
-
git push sends the changes to the remote repository (server)


Stages in Git
The following are the three stages in the Git workflow:
-
Working Area: You can edit files using your favorite editor/Integrated Development Environment (IDE).
-
Staging Area: You have made the changes and added the changes to Git. You can still make changes here. (From the analogy explained in the next card) It is like taking an item out of the box, where the box is the staging area. (
git add
) -
Local Repository: You have finalized the changes and committed them with a new hash and proper message. (
git commit
)
Remote Repository: You can now push the changes to online platforms like Github or Gitlab from where others can collaborate. (git push
)


Git Stages: Analogy
Git works in three stages known as The Three Trees
: Working Area, Staging Area, and Local Repository, which means Git maintains three states of a file. To understand this better, let us take an analogy of a box.
Assume you are packing items in the house in different boxes and labeling them to identify it later.
-
Get a table and keep all the items that you want to pack underneath. (
git init
) -
Now you might select specific kitchen items, dust them, and club similar items (like spoons) together. (doing changes – Working area)
-
Add the items that are ready to the box. (
git add
– Staged) -
Seal the box and add a label – ‘Kitchen Items’. (
git commit
– Committed)
After git commit
, a unique hash is created and the changes are saved.


Git Basic Commands Demo


Ignore or Keep
If you do not want Git to track any file/directory, you can add the file/directory to .gitignore
file. To track an empty directory, you need to add .gitkeep
to that empty directory, as Git ignores them by default.
This video explains how to do that.


Visualizing Git
To visualize the commands and see how your repository changes with time, you can try out Git commands in visualizing-git.
Cool Stuff Below !
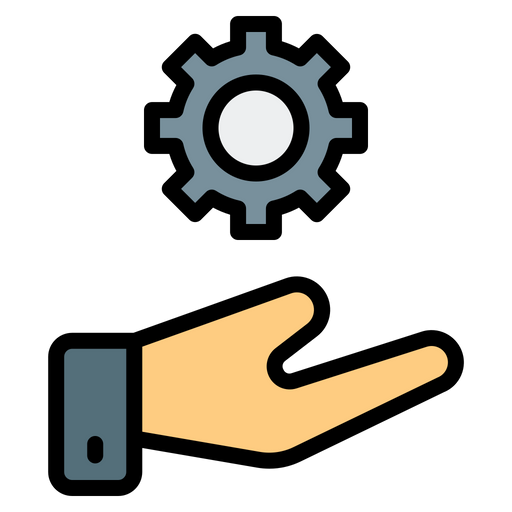
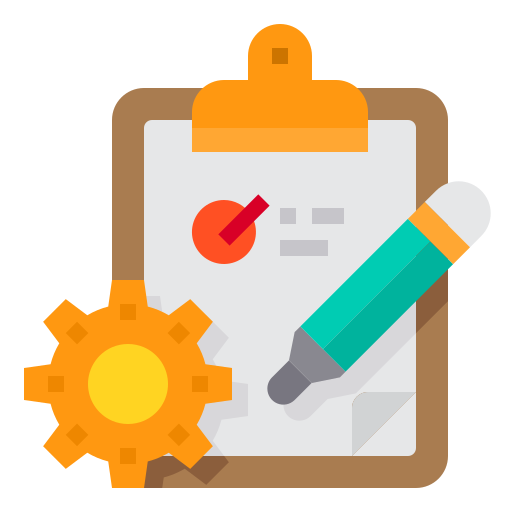
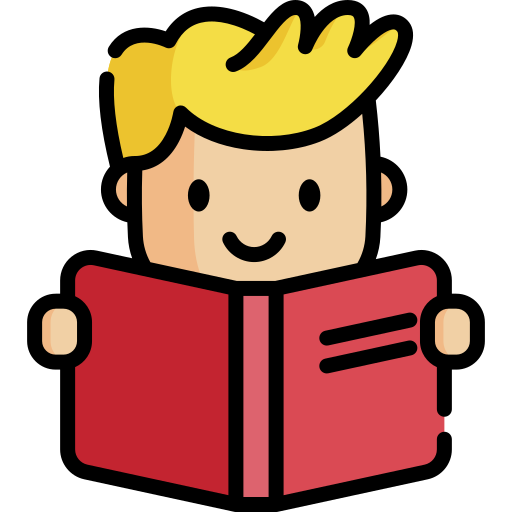